I've looked into this, using the following test code and the original Gunman asset:
require("mobdebug").start()
local spine = require "spine-corona.spine"
function loadSkeleton(atlasFile, jsonFile, x, y, scale, animation, skin)
local imageLoader = function (path)
local paint = { type = "image", filename = "data/" .. path }
return paint
end
local atlas = spine.TextureAtlas.new(spine.utils.readFile("data/" .. atlasFile), imageLoader)
local json = spine.SkeletonJson.new(spine.AtlasAttachmentLoader.new(atlas))
json.scale = scale
local skeletonData = json:readSkeletonDataFile("data/" .. jsonFile)
local skeleton = spine.Skeleton.new(skeletonData)
skeleton.scaleY = -1
skeleton.group.x = x
skeleton.group.y = y
if skin then skeleton:setSkin(skin) end
local animationStateData = spine.AnimationStateData.new(skeletonData)
animationStateData.defaultMix = 0.5
local animationState = spine.AnimationState.new(animationStateData)
return { skeleton = skeleton, state = animationState }
end
local lastTime = 0
local result = loadSkeleton("Gunman.atlas", "Gunman.json", 240, 300, 0.4, "Run")
local skeleton = result.skeleton
local state = result.state
state:setAnimationByName(0, "Run", true)
state:setAnimationByName(1, "Aim_Sniper", true)
local aimBone = skeleton:findBone("AimPivot")
display.setDefault("background", 0.2, 0.2, 0.2, 1)
Runtime:addEventListener("enterFrame", function (event)
local currentTime = event.time / 1000
local delta = currentTime - lastTime
lastTime = currentTime
aimBone.rotation = 45;
state:update(delta)
state:apply(skeleton)
skeleton:updateWorldTransform()
end)
Which gives the following expected result using the 3.7 runtimes:
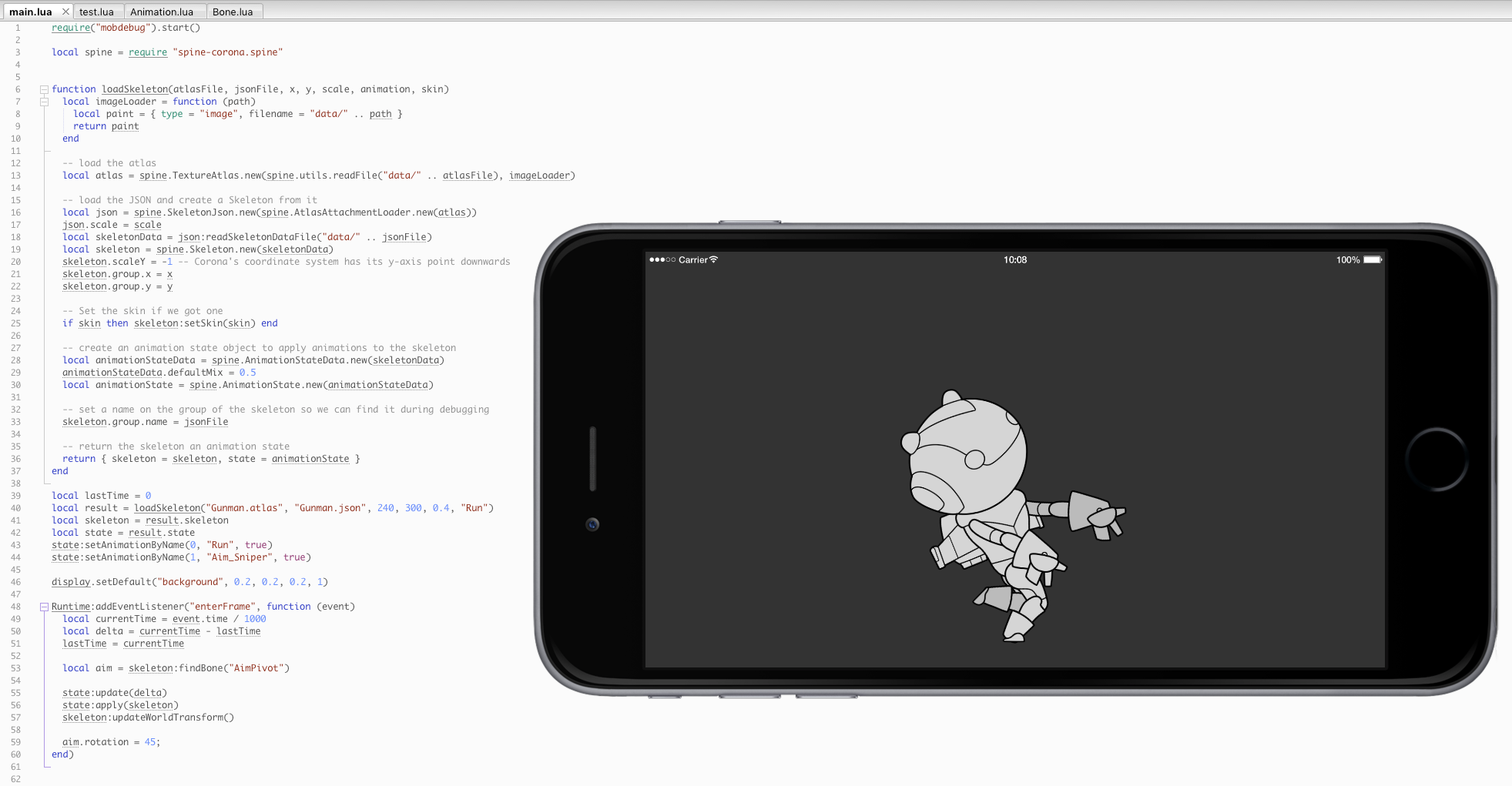
Please modify the above code to reproduce the issue.